Simple Java Program Structure :
The best way to learn a new language is to write a few simple example programs & execute them. To do that we have to know the structure of the Java program. More precisely, we have to know how to create a simple Java program and what its different sections mean. Let's take a very simple program as example, that prints a line of test as output.
class SampleOne
{
public static void main (String ergs[ ])
{
System.out.println("This is my first Java program");
}
}
Class Declaration :
The first line i,e "class SampleOne" declares a class, which is an object-oriented construct. As Java is a true object-oriented language, So everything must be placed inside a class. class is a keyword and declares that a new class definition follows. SampleOne is a java identifier that specifies the name of the class to be defined.
Braces :
Every class definition in Java begins with an opening braces "{" and ends with a matching closing braces "}". Basically, two curly brackets i,e {...} are used to group all the commands, so it is known that the commands belong to the specific class or method.
The main Line :
The third line i,e "public static void main (String args [ ])" defines a method named main. Every Java application program must include the main() method. This is the starting point for the interpreter to begin the execution of the program. A Java application can have any number of classes but only one of them must include main method to initiate the execution. This line contains a number of keywords, public, static and void.
public - The keyword public is an access specifier that declares the main method as unprotected and therefore making it accessible to all other classes.
static - It declares this method as one that belongs to the entire class and not a part of any objects of the class. The main must always be declared as static since the interpreter uses this method before any objects are created.
void - The type modifier void states that the main method doesn't return any value (but in this case simply prints some test to screen).
String args [ ] - All parameters to a method are declared inside a pair of parentheses. String args [ ] declares a parameter named args, which contains an array of objects of the class type String.
The Output Line :
The only executable statement in this program is System.out.println ("This is my first Java program"); This println method is a member of the out object, which is a static data member of System class. The method println always appends a newline character to the end of the string. This means any subsequently output will start on a new line. Every Java statement must end with a semicolon.
Java Program Structure:
A Java program may contain one or more sections as described below -
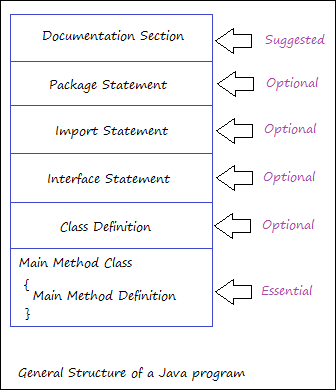
Documentation Section :
This section comprises a set of comment lines giving the name of the program, the author and other details, which the programmer would like to refer to at a larger stage. Comments must explain why & what of classes and how of algorithms. This would extremely help in maintaining the program. Java uses a third style of comment /**....*/ known as Documentation Comment. This form of comment is used for generating documentation automatically.
Package Statement :
The first statement allowed in a Java file is a package statement. This statement declares a package name and informs the compiler that the classes defined here belong to this package. So basically, a package is a group of classes that are defined by a name. This package statement is optional. We can declare package as follows -
package packageName;
Import Statements :
The next thing after a package statement (but before any classes definitions) may be a number of import statements. This statement instructs the interpreter to load the test class contained in the package, which has been imported. Using import statements, we can have access to classes that are part of a other named packages. We can write the import statement as below -
import packageName;
Interface Statement :
An interface is like a class but includes a group of method declarations. This is also an optional section and is used only when we wish to implement the multiple inheritance feature in the program.
Class Definition :
A Java program may contain multiple class definitions. Classes are the primary and essential elements of a Java program. These classes are used to map the objects of real-world problems. The number of classes used depends on the complexity of the problem. Classes are the main and essential elements of any Java program.
Main Method Class :
Since every Java stand-alone program requires a main method as it's starting point, this class is the essential part of a Java program. A simple Java program may contain only this part. The main method creates objects of various classes and establishes communications between them. Main method contains data type declaration and executable statements. On reaching the end of main method, the program terminates and the control passes back to the operating system.