Implementing a Java Program :
Implementation of a Java program involves a series of steps. They include -
Creating the program :
We can create a program using any test editor. In this example, we will use Notepad. it is a simple editor included with the Windows Operating System. You can use a different text editor like NotePad++ etc. Let's open the Notepad & type the following Java code -
package myFirstJavaProject;
import java.util.Scanner;
public class myFirstJavaProgram {
public static void main(String args[]) {
// Variable Initialization
String strSuccess = "Bravo!! Now you are a Java programmer";
String strFailure = "Unfortunately You are not successfull right now, but trust me You will :)";
System.out.println("Let's understand Java program step by step");
System.out.println("So, are you ready for it? Please type Yes or No");
Scanner answer = new Scanner(System.in);
String input = answer.nextLine();
if (input.equals("Yes")) {
System.out.println(strSuccess);
} else {
System.out.println(strFailure);
}
}
}
Remember that, before we begin compiling the program, the Java Development Kit (JDK) must be properly installed on your system.
We must save this program in a file called "myFirstJavaProgram.java" ensuring that the file name contains the class name properly. This file is called the source file. Source file will always have the extension java. If a program contains multiple classes, the file name must be the class name of the class containing the main method.
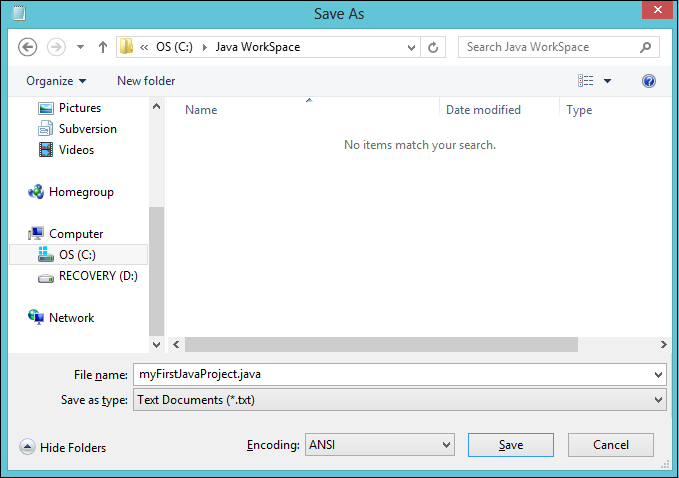
Compiling the program :
To compile the program, we must run the java compiler javac, with the name of the source file on the command line as shown below - javac myFirstJavaProgram.java If your program is error free then javac compiler creates a file called " myFirstJavaProgram.class" containing the bytecode of the program. Note that the compiler automatically names the bytecode file as <classname> .class.
a) Open the command prompt for the right path, where your executable java file is present.

b) Type javac <yourJavaFileName.java> and press Enter as shown in below image. In this example, the java file name is "myFirstJavaProgram".
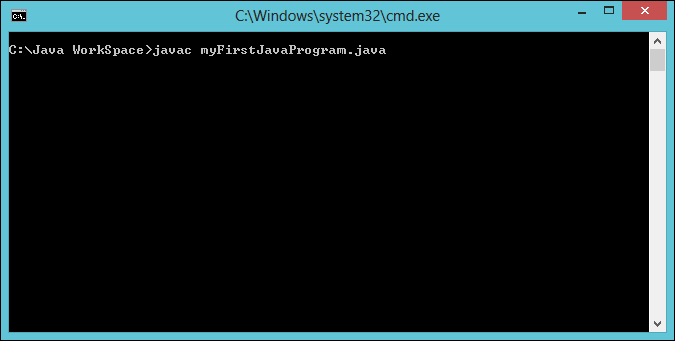
c) If your compilation is successful, if you look in your working folder, you can see that a file named myFirstJavaProgram.class has been created.
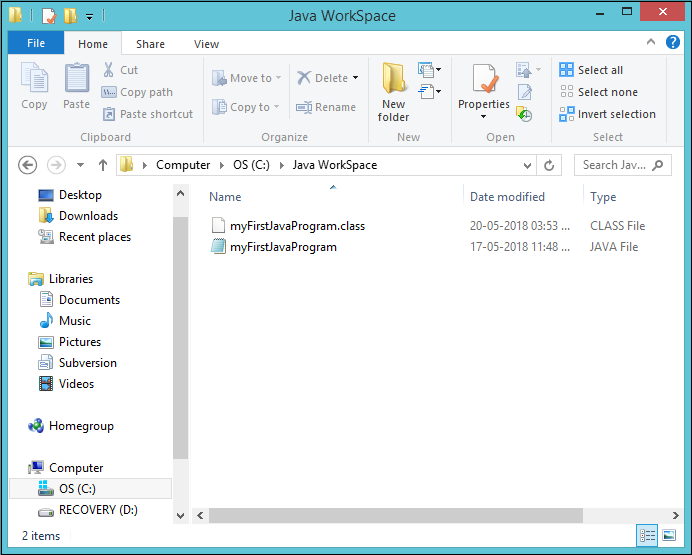
Running the Program:
We need to use the Java interpreter to run a stand-alone program. At the command prompt, we have to type as follows - java myFirstJavaProgram Now interpreter will look for the main method in the program and begins execution from there. When executed, our program displays the following output -